How to make HTTP requests in Node.js without installing external libraries
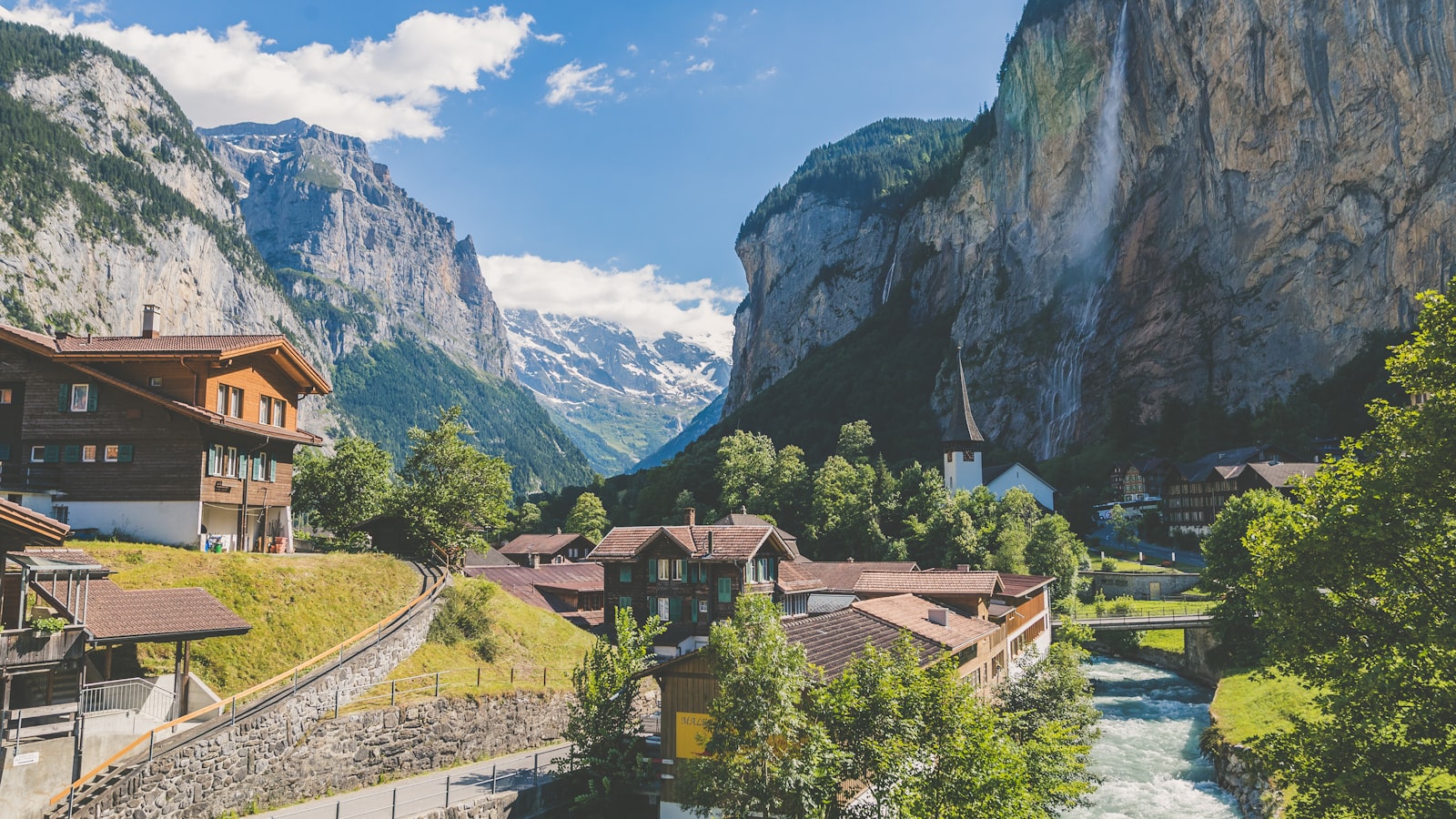
- Published on
- /3 mins read/---
When working with Node.js, there are many libraries that support creating requests to another server, such as node-fetch
, phin
, got
or request
(deprecated)...
However, if your server simply serves as an API for a client app and you now need to request
a JSON
file from another server or simply fetch an external API, which library should you choose to use?
The answer is that you don't need to add a new dependency to your package.json
just for creating a request because Node.js has a built-in module called https
.
HTTPS
https
is a lightweight module that comes pre-built in Node.js and is supported in most Node.js versions.
You can create a request
using https
as follows:
let https = require('https')
https
.get(url, (res) => {
let body = ''
res.on('data', (chunk) => (body += chunk))
res.on('end', () => {
try {
let json = JSON.parse(body)
// Now you can use json data...
} catch (err) {
console.error(`Failed to parse JSON data - ${err.toString()}`)
}
})
})
.on('error', (err) => {
console.error(`Failed to make request! Error: ${err.toString()}`)
})
In the code above:
- https is a built-in module in Node.js so you can
require
it directly without the need for installation. - You can create a request using
https.get(url[, options][, callback])
. - In the callback, you listen to the response events using
res.on()
. - Each time
res.on("data")
is triggered, you add thedata
to the stringbody
. - When
res.on("end")
is triggered, you can simply parse the body into a JSON object usingJSON.parse(body)
. - Lines
8
and11
: Note that you should parse the data within atry {} catch() {}
block to catch errors if the JSON cannot be parsed. - If the request fails, the
error
event will be triggered.
That's it! Now let's abstract this logic into a function that can be used anywhere in the server
:
let https = require('https')
let fetchJSON = (url) => {
return new Promise((resolve, reject) => {
https
.get(url, (res) => {
let body = ''
res.on('data', (chunk) => (body += chunk))
res.on('end', () => {
try {
resolve(JSON.parse(body))
} catch (err) {
reject(err)
}
})
})
.on('error', reject)
})
}
module.exports = fetchJSON
And you can use it as follows:
// Async context
let data = await fetchJSON(url)
// Use the data...
Good luck!